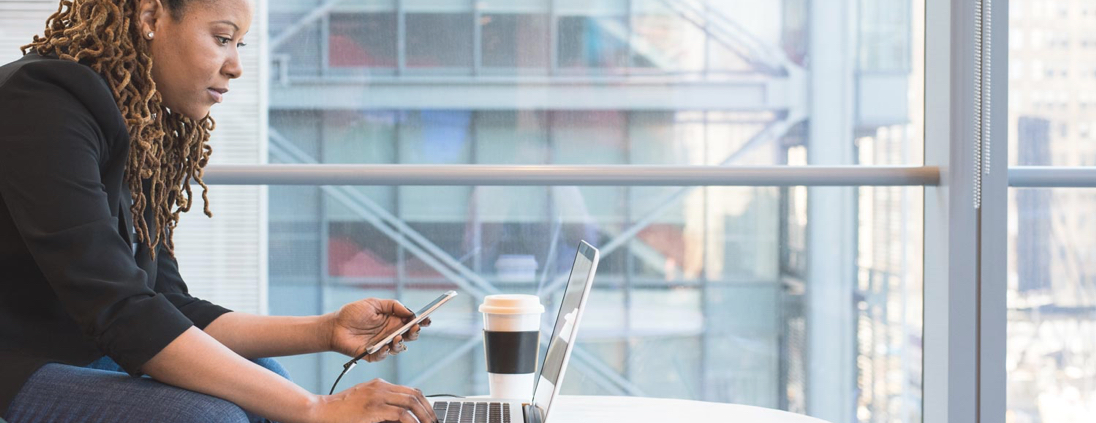
Nanodegree key: nd004-cn
Version: 1.0.0
Locale: en-us
Learn how to create server-side, data-driven web applications that support any front-end and can scale to support hundreds of thousands of users.
Content
Part 01 : Project - Movie Website
You will write server-side code to store a list of your favorite movies, including box art imagery and a movie trailer URL. You will then serve this data as a web page allowing visitors to review their movies and watch the trailers.
-
Module 01: Welcome to the Nanodegree
-
Lesson 01: Welcome to the Nanodegree
- Concept 01: Welcome to the Full Stack Nanodegree
- Concept 02: Welcome to Orientation!
- Concept 03: Projects and Progress
- Concept 04: Connecting with Your Community
- Concept 05: Support from the Udacity Team
- Concept 06: How Does Project Submission Work?
- Concept 07: Integrity and Mindset
- Concept 08: How Do I Find Time for My Nanodegree?
- Concept 09: Finding Time to Study
-
-
Module 02: Programming Foundations with Python
-
Lesson 01: Introductions
Review programming fundamentals such as loops and conditionals, and get prepared for your journey deeper into programming.
-
Lesson 02: Use functions
Use Python functions to build two mini-projects: a break timer that interacts with the web browser, and a program to hide a secret message in a collection of files.
- Concept 01: Course Map
- Concept 02: Take a Break (Story)
- Concept 03: Take a Break (Output)
- Concept 04: How Would You Do This?
- Concept 05: Planning the Break
- Concept 06: One Way of Doing This
- Concept 07: Launching Python
- Concept 08: What Is the Error?
- Concept 09: Squashing the Bug
- Concept 10: Making the Program Wait
- Concept 11: Adding a Loop
- Concept 12: Making the Program Wait Longer
- Concept 13: Where Does Webbrowser Come From?
- Concept 14: Reading Webbrowser Documentation
- Concept 15: Take a Break Mini-Project
- Concept 16: Course Map
- Concept 17: Secret Message (Story)
- Concept 18: Secret Message (Output)
- Concept 19: Planning a Secret Message
- Concept 20: Opening a File
- Concept 21: Changing Filenames
- Concept 22: Checking os Documentation
- Concept 23: Renaming Files
- Concept 24: What Is the Error?
- Concept 25: Squashing the Bug
- Concept 26: Rename Troubles
- Concept 27: Where Does os Come From?
- Concept 28: Reading os Documentation
- Concept 29: Secret Message Mini-Project
- Concept 30: Edge Case
- Concept 31: When Functions Do Not Suffice
-
Lesson 03: Use classes: Draw Turtles
Learn and apply object-oriented programming (OOP) by using Python's turtle graphics library to draw shapes in a graphical interface.
- Concept 01: Course Map
- Concept 02: Drawing Turtles (Story)
- Concept 03: Drawing Turtles (Output)
- Concept 04: How to Draw a Square
- Concept 05: Drawing a Square
- Concept 06: Change Turtle Shape, Color, and Speed
- Concept 07: Where Does Turtle Come From?
- Concept 08: Reading Turtle Documentation
- Concept 09: Two Turtles
- Concept 10: What's Wrong With This Code?
- Concept 11: Improving Code Quality
- Concept 12: What Is a Class?
- Concept 13: Making a Circle out of Squares
- Concept 14: Turtle Mini-Project
- Concept 15: Comfort Level
- Concept 16: They Look So Similar
-
Lesson 04: Use classes: Send Text
Advance your use of Python classes in a mini-project: sending text messages to mobile phones via the Twilio service.
- Concept 01: Course Map
- Concept 02: Send Text Messages (Story)
- Concept 03: Send Text Messages (Output)
- Concept 04: Introducing Twilio
- Concept 05: Download Twilio
- Concept 06: Twilio Download Feedback
- Concept 07: Setting Up Our Code
- Concept 08: Registering with Twilio
- Concept 09: Running Our Code
- Concept 10: Python Keyword From
- Concept 11: Investigating the Code
- Concept 12: Where Does Twilio Come From?
- Concept 13: Connecting Turtle and Twilio
- Concept 14: Send Text Messages Mini-Project
-
Lesson 05: Use classes: Profanity Editor
Plan and build a program using advanced Python functions and classes to save your instructor from an embarrassing typo!
- Concept 01: Course Map
- Concept 02: Embarrassing Story
- Concept 03: Planning Profanity Editor
- Concept 04: Reading from a File
- Concept 05: Place Function Open
- Concept 06: Reading Open Documentation
- Concept 07: Connecting Turtle and Open
- Concept 08: Built-In Python Functions
- Concept 09: Checking for Curse Words
- Concept 10: Accessing a Website with Code
- Concept 11: Place urllib and urlopen
- Concept 12: Reading urllib Documentation
- Concept 13: Printing a Better Output
- Concept 14: Profanity Editor Mini-Project
- Concept 15: Connecting Turtle, Open, and Urllib
-
Lesson 06: Make classes: Movie Website
Apply Python classes to build a website to share images or trailers of your favorite movies.
- Concept 01: Course Map
- Concept 02: What Should Class Movie Remember?
- Concept 03: Defining Class Movie
- Concept 04: What Happens When
- Concept 05: Defining __init__
- Concept 06: What Is Going On Behind the Scenes
- Concept 07: What Will Be the Output?
- Concept 08: Behind the Scenes
- Concept 09: Is Self Important?
- Concept 10: Next Up: Show_trailer
- Concept 11: Playing Movie Trailer
- Concept 12: Play Your Favorite Trailer
- Concept 13: Recap Vocab
- Concept 14: Designing the Movie Website
- Concept 15: Coding the Movie Website
- Concept 16: Movie Website Mini-Project
- Concept 17: Comfort Level
-
Lesson 07: Make classes: Advanced Topics
Explore advanced topics in Python OOP, such as class variables, inheritance, and method overriding.
- Concept 01: Advanced Ideas in OOP
- Concept 02: Class Variables
- Concept 03: Using Predefined Class Variables
- Concept 04: Inheritance
- Concept 05: Class Parent
- Concept 06: What's the Output?
- Concept 07: Class Child
- Concept 08: Transitioning to Class Movie
- Concept 09: Updating the Design for Class Movie
- Concept 10: Reusing Methods
- Concept 11: Method Overriding
- Concept 12: Next Stop - Final Project
-
-
Module 03: Project: Movie Website
-
Lesson 01: Movie Trailer Website
You will write server-side code to store a list of your favorite movies, including box art imagery and a movie trailer URL.
-
Part 02 : Project - Build a Portfolio Site
You will be provided with a design mockup as a PDF-file and must replicate that design in HTML and CSS. You will develop a responsive website that will display images, descriptions and links to each of the portfolio projects you will complete throughout the course of the Front-End Web Developer Nanodegree.
-
Module 01: Intro to HTML & CSS
-
Lesson 01: HTML Syntax
Set up your development environment for writing HTML, learn about HTML tree structure and write HTML syntax.
- Concept 01: Lesson Introduction
- Concept 02: HTML Structure Part 1
- Concept 03: Environments
- Concept 04: Text Editors
- Concept 05: Browsers
- Concept 06: Workflow
- Concept 07: Trees
- Concept 08: HTML and Trees
- Concept 09: A Guide to Paths
- Concept 10: Constructing Links
- Concept 11: HTML Forms
- Concept 12: HTML Structure Part 2
- Concept 13: HTML Documents in Depth
- Concept 14: HTML Syntax Outro
-
Lesson 02: CSS Syntax
Get started with the basics of CSS, unleash the power of developer tools to inspect webpages and apply changes on the fly,
use CSS units, colors and fonts, and start adding style to your website.- Concept 01: Getting Started with CSS
- Concept 02: What is CSS?
- Concept 03: CSS Rulesets
- Concept 04: Comments
- Concept 05: Attributes and Selectors
- Concept 06: Developer Tools
- Concept 07: Developer Tools on Different Browsers
- Concept 08: Quiz: Using Developer Tools
- Concept 09: CSS Units
- Concept 10: CSS Colors
- Concept 11: Style an Image
- Concept 12: Style the Font
- Concept 13: What is a Stylesheet?
- Concept 14: Outro
-
Lesson 03: Sizing
Use the box model to size and position elements.
- Concept 01: Guess the Render Game
- Concept 02: James' Solution
- Concept 03: Cameron's Solution
- Concept 04: Who's the Winner?
- Concept 05: Boxes, Boxes Everywhere
- Concept 06: Boxes on the Web
- Concept 07: The Box Model
- Concept 08: Border-Box
- Concept 09: Changing Boxes
- Concept 10: Box Model Sizing
- Concept 11: Containers
- Concept 12: Relative Widths
- Concept 13: Inline Boxes
- Concept 14: Semantic Elements
- Concept 15: Types of Elements
- Concept 16: Element Research
- Concept 17: Outro
-
Lesson 04: Positioning
Position elements using different flows.
- Concept 01: Intro
- Concept 02: Normal Flow
- Concept 03: Normal Flow Quiz
- Concept 04: Inline-Block
- Concept 05: Why Two Lines?
- Concept 06: Anonymous Boxes
- Concept 07: Relative Flow
- Concept 08: Relative Flow Quiz
- Concept 09: Relative Neighbors
- Concept 10: 3D Websites
- Concept 11: Document and Viewport
- Concept 12: Fixed Flow
- Concept 13: Fixed Flow Quiz
- Concept 14: Absolute Flow
- Concept 15: Inline Formatting
- Concept 16: Debugging CSS Part 1
- Concept 17: Debugging CSS Part 2
- Concept 18: Debugging CSS Solution
- Concept 19: Outro
-
Lesson 05: Floats
Use floats to extend and improve your ability to create layouts.
-
-
Module 02: Responsive Design
-
Lesson 01: Why Responsive?
Explore the case for responsive design. You'll see how the experience of using the web changes from one device type to another.
- Concept 01: Sites On Mobile
- Concept 02: Intro to Project
- Concept 03: Pan, Zoom, Touch, Ick
- Concept 04: Emulators, Simulators and Real Devices
- Concept 05: Setting up Chrome's Dev Tools
- Concept 06: Remote Debugging Intro
- Concept 07: Setup for Mobile
- Concept 08: Using Dev Tools on Mobile
- Concept 09: Mobile Tools for iOS
- Concept 10: Lesson Summary
-
Lesson 02: Starting Small
Use the best practices in responsive design to start building from the smallest devices (mobile phones) up to the largest displays (desktop computers).
- Concept 01: Defining the Viewport
- Concept 02: Pixels, pixels and moar pixels!
- Concept 03: Setting the Viewport
- Concept 04: Setting the Viewport
- Concept 05: Large Fixed Width Elements
- Concept 06: Max-width on elements
- Concept 07: Tap Target Sizes
- Concept 08: Start Small
- Concept 09: Project Solution - Long
- Concept 10: Lesson Summary
-
Lesson 03: Building Up
Use media queries to define breakpoints in your application to easily split your site into different versions based on display size.
- Concept 01: Lesson Intro
- Concept 02: Basic Media Query Intro
- Concept 03: Adding a Basic Media Query
- Concept 04: Adding a basic media query 2
- Concept 05: Next Step Media Queries
- Concept 06: What Styles Are Applied?
- Concept 07: Breakpoints
- Concept 08: Breakpoints Pt. II
- Concept 09: Picking Breakpoints
- Concept 10: Picking Breakpoints 2
- Concept 11: Complex Media Queries
- Concept 12: Grids
- Concept 13: Flexbox Intro
- Concept 14: Flexbox Container
- Concept 15: Flex Item
- Concept 16: Deconstructing a Flexbox Layout
- Concept 17: Responsive Design Outro
-
Lesson 04: Common Responsive Patterns
Walk through the most popular responsive layout patterns and learn the tools needed to implement them in your own designs.
- Concept 01: Intro to Patterns
- Concept 02: Pattern - Column Drop
- Concept 03: Pattern - Mostly Fluid
- Concept 04: Mostly Fluid Part 1
- Concept 05: Mostly Fluid Part 2
- Concept 06: Combining Fluid Layouts
- Concept 07: Pattern - Layout Shifter
- Concept 08: Which is Which?
- Concept 09: Pattern - Off Canvas
- Concept 10: Off Canvas Visualization
- Concept 11: Project Update Part 2
- Concept 12: Lesson Summary
-
Lesson 05: Optimizations
Learn how to optimize images, tables, and fonts to make for the best responsive layouts.
- Concept 01: Lesson Intro
- Concept 02: Images
- Concept 03: Responsive Tables Intro
- Concept 04: Responsive Tables - Hidden Columns
- Concept 05: Hide Some Columns
- Concept 06: Responsive Tables - No More Tables
- Concept 07: Responsive Tables - Contained Scrolling
- Concept 08: Fonts
- Concept 09: Minor Breakpoints
- Concept 10: Final Project Updates
- Concept 11: Wrap Up
-
-
Module 03: Build a Portfolio Site
-
Lesson 01: Build a Portfolio Site
Given a pdf mockup of a website from a designer, translate it to a real website using your HTML and CSS skills.
-
Part 03 : Developers' Tools
Brush up your knowledge of essential developers' tools such as the Unix shell, Git, and Github; then apply your skills to investigate HTTP, the Web's fundamental protocol.
-
Module 01: Shell Workshop
-
Lesson 01: Shell Workshop
The Unix shell is a powerful tool for developers of all sorts. In this lesson, you'll get a quick introduction to the very basics of using it on your own computer.
- Concept 01: The Command Line
- Concept 02: Intro to the Shell
- Concept 03: Windows: Installing Git Bash
- Concept 04: Opening a terminal
- Concept 05: Your first command (echo)
- Concept 06: Navigating directories (ls, cd, ..)
- Concept 07: Current working directory (pwd)
- Concept 08: Parameters and options (ls -l)
- Concept 09: Organizing your files (mkdir, mv)
- Concept 10: Downloading (curl)
- Concept 11: Viewing files (cat, less)
- Concept 12: Removing things (rm, rmdir)
- Concept 13: Searching and pipes (grep, wc)
- Concept 14: Shell and environment variables
- Concept 15: Startup files (.bash_profile)
- Concept 16: Controlling the shell prompt ($PS1)
- Concept 17: Aliases
- Concept 18: Keep learning!
-
-
Module 02: Version Control with Git & GitHub
-
Lesson 01: What is Version Control?
Version control is an incredibly important part of a professional programmer's life. In this lesson, you'll learn about the benefits of version control and install the version control tool Git!
-
Lesson 02: Create A Git Repo
Now that you've learned the benefits of Version Control and gotten Git installed, it's time you learn how to create a repository.
-
Lesson 03: Review a Repo's History
Knowing how to review an existing Git repository's history of commits is extremely important. You'll learn how to do just that in this lesson.
-
Lesson 04: Add Commits To A Repo
A repository is nothing without commits. In this lesson, you'll learn how to make commits, write descriptive commit messages, and verify the changes you're about to save to the repository.
-
Lesson 05: Tagging, Branching, and Merging
Being able to work on your project in isolation from other changes will multiply your productivity. You'll learn how to do this isolated development with Git's branches.
-
Lesson 06: Undoing Changes
Help! Disaster has struck! You don't have to worry, though, because your project is tracked in version control! You'll learn how to undo and modify changes that have been saved to the repository.
-
Lesson 07: Working With Remotes
You'll learn how to create remote repositories on GitHub and how to get and send changes to the remote repository.
-
Lesson 08: Working On Another Developer's Repository
In this lesson, you'll learn how to fork another developer's project. Collaborating with other developers can be a tricky process, so you'll learn how to contribute to a public project.
-
Lesson 09: Staying In Sync With A Remote Repository
You'll learn how to send suggested changes to another developer by using pull requests. You'll also learn how to use the powerful
git rebase
command to squash commits together.
-
-
Module 03: HTTP & Web Servers
-
Lesson 01: Requests & Responses
In this lesson, you will examine HTTP requests and responses by experimenting directly with a web server, interacting with it by hand.
-
Lesson 02: The Web from Python
In this lesson, you will write HTTP servers and clients in Python.
- Concept 01: Intro
- Concept 02: Python's http.server
- Concept 03: What about `.encode()`?
- Concept 04: The echo server
- Concept 05: Queries and quoting
- Concept 06: HTML and forms
- Concept 07: GET and POST
- Concept 08: A server for POST
- Concept 09: Post-Redirect-Get
- Concept 10: Making requests
- Concept 11: Using a JSON API
- Concept 12: The bookmark server
- Concept 13: You did it!
-
Lesson 03: HTTP in the Real World
In this lesson, you will examine a number of practical HTTP features that go beyond basic requests and responses.
-
Part 04 : Project - Logs Analysis
You will analyze data from the logs of a web service to answer questions such as "What is the most popular page?" and "When was the error rate high?" using advanced SQL queries.
-
Module 01: Relational Databases
-
Lesson 01: Data and Tables
Learn the principles behind relational data organization: tables, queries, aggregations, keys, and joins.
- Concept 01: Welcome to Relational Databases
- Concept 02: What's a Database
- Concept 03: Looking at Tables
- Concept 04: Data Types and Meaning
- Concept 05: Data Meanings
- Concept 06: Zoo
- Concept 07: Anatomy of a Table
- Concept 08: Answering Questions from a Table
- Concept 09: Aggregations
- Concept 10: Queries and Results
- Concept 11: How Queries Happen
- Concept 12: Favorite Animals
- Concept 13: Related Tables
- Concept 14: Uniqueness and Keys
- Concept 15: Primary Key
- Concept 16: Joining Tables
- Concept 17: Database Concepts
- Concept 18: Summary
-
Lesson 02: Elements of SQL
Start learning SQL by using the select and insert statements to read and write data in database tables.
- Concept 01: SQL is for Elephants
- Concept 02: Talk to the Zoo Database
- Concept 03: Types in the SQL World
- Concept 04: Just a few SQL types
- Concept 05: Select Where
- Concept 06: Comparison Operators
- Concept 07: The One Thing SQL is Terrible At
- Concept 08: The Experiment Page
- Concept 09: Select Clauses
- Concept 10: Why Do It in the Database
- Concept 11: Count All the Species
- Concept 12: Insert: Adding Rows
- Concept 13: Find the Fish-Eaters
- Concept 14: After Aggregating
- Concept 15: More Join Practice
- Concept 16: Wrap up
- Concept 17: Installing the Virtual Machine
- Concept 18: Reference — Elements of SQL
-
Lesson 03: Python DB-API
Learn the Python database API, and apply your knowledge to fix common bugs that arise in database-backed web services.
- Concept 01: Welcome to your Database
- Concept 02: What's a DB-API
- Concept 03: Writing Code with DB API
- Concept 04: Trying out DB API
- Concept 05: Inserts in DB API
- Concept 06: Running the Forum
- Concept 07: Hello PostgreSQL
- Concept 08: Give That App a Backend
- Concept 09: Bobby Tables, Destroyer of Posts
- Concept 10: Curing Bobby Tables
- Concept 11: Spammy Tables
- Concept 12: Stopping the Spam
- Concept 13: Updating Away the Spam
- Concept 14: Deleting the Spam
- Concept 15: Conclusion
- Concept 16: Reference — Python DB-API
-
Lesson 04: Deeper Into SQL
Create your own database tables using normalized table design, using keys to declare relationships between tables; then apply these relationships to draw conclusions from data.
- Concept 01: Intro to Creating Tables
- Concept 02: Normalized Design Part One
- Concept 03: Normalized Design Part Two
- Concept 04: What's Normalized
- Concept 05: Create Table and Types
- Concept 06: Creating and Dropping
- Concept 07: Declaring Primary Keys
- Concept 08: Declaring Relationships
- Concept 09: Foreign Keys
- Concept 10: Self Joins
- Concept 11: Counting What Isn't There
- Concept 12: Subqueries
- Concept 13: One Query Not Two
- Concept 14: Views
- Concept 15: Reference — Deeper into SQL
- Concept 16: Outro
-
Lesson 05: Logs Analysis
In this project, you'll practice your SQL skills by building a reporting tool that summarizes data from a large database.
-
Part 05 : Project - Item Catalog
You will develop an application that provides a list of items within a variety of categories as well as provide a user registration and authentication system. Registered users will have the ability to post, edit and delete their own items.
-
Module 01: Full Stack Foundations
-
Lesson 01: Working with CRUD
Learn the CRUD pattern (Create, Read, Update, Delete) and how it relates to RESTful architectures and to the operations of a database-backed web service.
- Concept 01: Course Intro
- Concept 02: Prerequisites & Preparation
- Concept 03: Project Introduction - CRUD
- Concept 04: CRUD Review 1
- Concept 05: CRUD Review 2
- Concept 06: CRUD Review 3
- Concept 07: CRUD Review 4
- Concept 08: SQL
- Concept 09: SQL Quiz Select
- Concept 10: SQL Insert Into
- Concept 11: SQL Update
- Concept 12: SQL Delete
- Concept 13: Creating a Database and ORMs
- Concept 14: Introducing SQLAlchemy
- Concept 15: Creating a Database - Configuration
- Concept 16: Creating a Database - Class and Table
- Concept 17: Creating a Database - Mapper
- Concept 18: Putting It All Together
- Concept 19: Database Setup Quiz Part 1
- Concept 20: Database Setup Quiz Part 2
- Concept 21: CRUD Create
- Concept 22: CRUD Create Quiz
- Concept 23: CRUD Read
- Concept 24: CRUD Read Quiz
- Concept 25: CRUD Update
- Concept 26: CRUD Update Quiz
- Concept 27: CRUD Delete
- Concept 28: CRUD Delete Quiz
- Concept 29: CRUD Review
- Concept 30: Wrap Up
-
Lesson 02: Making a Web Server
Build a web service in Python that uses your database to implement CRUD operations.
- Concept 01: Lesson 2 Introduction
- Concept 02: Review of Clients, Servers and Protocols
- Concept 03: HTTP and Response Codes
- Concept 04: Building a Server with HTTPBaseServer
- Concept 05: Running a Web Server
- Concept 06: Port Forwarding
- Concept 07: Responding to Multiple GET Requests
- Concept 08: Hola Server
- Concept 09: Adding POST to web server
- Concept 10: Running the POST Web Server
- Concept 11: Adding CRUD to our Website
- Concept 12: CRUD Objectives
- Concept 13: Adding CRUD Hints
- Concept 14: CRUD Hints
- Concept 15: Objective 1
- Concept 16: Objective 2
- Concept 17: Objective 3
- Concept 18: Objective 4
- Concept 19: Objective 5
- Concept 20: Lesson 2 Wrap-Up
-
Lesson 03: Developing with frameworks
Apply the Flask framework in Python to build web services more quickly and reliably.
- Concept 01: Introducing Frameworks and Flask
- Concept 02: Lesson 3 Overview
- Concept 03: Running Your First Flask Application
- Concept 04: First Flask App Quiz
- Concept 05: Adding Database to Flask Application
- Concept 06: Adding Database to Flask Application Quiz
- Concept 07: Routing
- Concept 08: Routing Create Quiz
- Concept 09: Templates
- Concept 10: Templates Quiz
- Concept 11: URL for Quiz
- Concept 12: Form Requests and Redirects
- Concept 13: Edit Menu Item Form Quiz
- Concept 14: Delete Menu Item
- Concept 15: Message Flashing
- Concept 16: Message Flashing Quiz
- Concept 17: Styling
- Concept 18: Responding With JSON
- Concept 19: JSON Quiz
- Concept 20: Lesson 3 Wrap-Up
-
Lesson 04: Iterative Development
Learn the basics of agile and iterative development while building a restaurant menu application.
- Concept 01: Lesson 4 Introduction
- Concept 02: Iterative Development
- Concept 03: Tackling a Complex Project
- Concept 04: Mockups Exercise
- Concept 05: Adding Routes
- Concept 06: Adding Templates and Forms
- Concept 07: CRUD Functionality
- Concept 08: API Endpoints
- Concept 09: Styling Your App
- Concept 10: Wrap-Up
-
-
Module 02: Authentication and Authorization
-
Lesson 01: Authentication vs Authorization
Learn the difference between authentication and authorization and some best practices in developing a login system.
- Concept 01: Intro
- Concept 02: Course Map
- Concept 03: Authentication
- Concept 04: Authentication is hard
- Concept 05: Implementing Authentication is Hard
- Concept 06: Using Third Party Auth Providers
- Concept 07: Authorization
- Concept 08: Authentication without Authorization
- Concept 09: Auth providers
- Concept 10: Pros and Cons using Third Party
- Concept 11: Follow the Flow
- Concept 12: Outro
-
Lesson 02: Creating Google Sign in
Investigate OAuth and build third-party sign-in into your web applications using Google's authentication services.
- Concept 01: Intro to Lesson 2
- Concept 02: Types of Flow
- Concept 03: Google+ Auth for server side apps
- Concept 04: Step 0 Get initial app running
- Concept 05: Step 1 Create Client ID & Secret
- Concept 06: Step 2 Create anti forgery state token
- Concept 07: Step 3 Create login page
- Concept 08: Step 4 Make a Callback Method
- Concept 09: Step 5 GConnect
- Concept 10: Step 6 Disconnect
- Concept 11: Step 7 Protecting Pages
- Concept 12: Wrap-up
-
Lesson 03: Local Permission System
Use a local permission system to protect pages based on each user, not just whether that user is logged in.
-
Lesson 04: Adding Facebook and other providers
Discover additional OAuth providers and add Facebook authentication in your application, giving more choices for third-party auth.
- Concept 01: Adding Additional OAuth Providers
- Concept 02: Registering your App with Facebook
- Concept 03: Client-Side Login with Facebook SDK
- Concept 04: Updating login.html
- Concept 05: Update project.py (part I)
- Concept 06: Update project.py (part II)
- Concept 07: Updating project.py Code
- Concept 08: Exploring other OAuth2.0 Providers
- Concept 09: Outro
-
-
Module 03: RESTful APIs
-
Lesson 01: What's and Why's of APIs
Discover the foundation of Web APIs, the common systems for passing and updating information to web applications.
- Concept 01: Course Intro
- Concept 02: Prerequisites
- Concept 03: What are APIs
- Concept 04: Web APIs
- Concept 05: Web API Protocols
- Concept 06: Digging into The Application Layer
- Concept 07: The Web Service Layer
- Concept 08: Content Formatting Layer
- Concept 09: Choosing the Right Technologies Soap vs Rest
- Concept 10: XML vs JSON
- Concept 11: Developer Discussions
- Concept 12: Developer Discussions
- Concept 13: Developer Discussions
- Concept 14: REST Constraints
- Concept 15: Why Stateless?
- Concept 16: Lesson 1 Wrap Up
-
Lesson 02: Accessing Published APIs
Practice gathering and reading information from web APIs that already live on the internet.
- Concept 01: Lesson 2 Intro
- Concept 02: Parts of an HTTP Request
- Concept 03: HTTP Response
- Concept 04: Sending API Requests with Postman and Curl
- Concept 05: Sending API Requests
- Concept 06: Searching for API Documentation
- Concept 07: Delving Into APIs
- Concept 08: Using the Foursquare API
- Concept 09: Requesting From Python Code
- Concept 10: Parsing Your Response
- Concept 11: Make Your Own API Mashup
- Concept 12: Lesson 2 Wrap Up
-
Lesson 03: Creating your own API Endpoints
Begin creating your own API endpoints and learn to serialize your data to package it up for transfer across the web using HTTP.
-
Lesson 04: Securing your API
Control access to your APIs by limiting who can access the resources behind them and ensuring that only authorized users can read and modify data.
- Concept 01: Lesson 4 Intro
- Concept 02: Adding Users and Logins
- Concept 03: User Registration
- Concept 04: Password Protecting a Resource
- Concept 05: Mom & Pop’s Bagel Shop
- Concept 06: Token Based Authentication
- Concept 07: Implementing Token Based Authentication in Flask
- Concept 08: Regal Tree Foods
- Concept 09: OAuth 2.0
- Concept 10: Adding OAuth 2.0 for Authentication
- Concept 11: Pale Kale Salads & Smoothies
- Concept 12: Rate Limiting
- Concept 13: Rate Limiting Exercise
- Concept 14: Bargain Mart
- Concept 15: Lesson 4 Wrap Up
-
Lesson 05: Writing Developer-Friendly APIs
Customize your APIs to be usable and approachable by the developers who will consume them.
-
-
Module 04: Project: Item Catalog
-
Lesson 01: Build an Item Catalog
You will develop an application that provides a list of items within a variety of categories as well as provide a user registration and authentication system. Registered users will have the ability to post, edit and delete their own items.
-
Part 06 : Project - Neighborhood Map
Extend the power of the web frontend using JavaScript, JQuery, and AJAX to build advanced interactive web applications.
-
Module 01: Intro to AJAX
-
Lesson 01: Requests and APIs
Learn how to request data from third party APIs using jQuery's AJAX functions. Examine AJAX queries in live applications and investigate APIs you can use in your own!
- Concept 01: Pre-Introduction
- Concept 02: Intro
- Concept 03: Client Server Demonstration
- Concept 04: AJAX Definition
- Concept 05: Asynchronous vs Synchronous Requests
- Concept 06: Facebook Example 1
- Concept 07: Facebook Example 2
- Concept 08: Facebook's AJAX Request
- Concept 09: Twitter Quiz
- Concept 10: Necessary components of an AJAX request
- Concept 11: API Inspiration
- Concept 12: Finding Examples of API Use
- Concept 13: API Brainstorm
- Concept 14: Download the Project
-
Lesson 02: Building the Move Planner App
Follow along as Cameron uses The New York Times API to build a moving planner app. Learn how to handle errors and how to debug your AJAX methods.
- Concept 01: Requests with jQuery
- Concept 02: Loading Streetview
- Concept 03: NYT API Key
- Concept 04: NYT Implementation
- Concept 05: Error Handling with NY Times
- Concept 06: CORS
- Concept 07: Wikipedia API
- Concept 08: Error Handling with JSON P
- Concept 09: Debugging
- Concept 10: Speeding up the First Render
- Concept 11: Finish
-
-
Module 02: JavaScript Design Patterns
-
Lesson 01: Changing Expectations
Learn why well-structured code is vitally important to a web app's structure, especially as the app gets larger. Explore how you can use MV* organizational framework to create cleaner projects.
- Concept 01: Welcome
- Concept 02: Introduce Cat Clicker and Andy
- Concept 03: Cat Clicker Requirements
- Concept 04: Reflections
- Concept 05: Andy's Reflections
- Concept 06: Requirements Change All The Time
- Concept 07: First Requirements Change
- Concept 08: Cat Clicker Requirements 2
- Concept 09: Reflections 2
- Concept 10: Andy's Reflections 2
- Concept 11: Closures and Event Listeners
- Concept 12: Second Requirements Change
- Concept 13: Cat Clicker Premium Requirements
- Concept 14: Reflections 3
- Concept 15: Andy's Reflections 3
- Concept 16: Spaghetti Code Story Time
- Concept 17: What is Spaghetti Code
- Concept 18: Introduction to MVO
- Concept 19: Explore The App's Structure
- Concept 20: Model Quiz
- Concept 21: View Quiz
- Concept 22: What Is the Model in Our Code
- Concept 23: What Is the View in Our Code
- Concept 24: What Is the Octopus in Our Code
- Concept 25: Identify the MVO in New App
- Concept 26: Where Should This Feature Live?
- Concept 27: Implement Note Date
- Concept 28: Segue Into L2
-
Lesson 02: Refactoring with Separation of Concerns
Begin refactoring your Cat Clicker code and learn the best ways to improve its structure.
- Concept 01: Introduction to Lesson 2
- Concept 02: Review Model and View for CC Premium
- Concept 03: Identify Octopus
- Concept 04: In Defense of Andy
- Concept 05: Rebuild Cat Clicker Premium
- Concept 06: Cat Clicker Premium Specs
- Concept 07: Cat Clicker Premium Solution
- Concept 08: Cat Clicker Premium Solution Review
- Concept 09: Cat Clicker Premium Pro
- Concept 10: Cat Clicker Premium Pro Specs
- Concept 11: How to Modernize Projects
- Concept 12: Interview with Nic
- Concept 13: Interview with Jacques
- Concept 14: Refactor Spaghetti Code
- Concept 15: Repository for Attendance App
- Concept 16: Interview With The Author
- Concept 17: Refactoring the Resumé
- Concept 18: Repository Information for Resume
- Concept 19: Segue Into L3
-
Lesson 03: Using an Organization Library
Learn about MV* frameworks like KnockoutJS to improve your Cat Clicker Application.
- Concept 01: MVO in the wild
- Concept 02: Library vs Framework 1
- Concept 03: Library vs Framework 2
- Concept 04: Interview with Nic About Using Libraries
- Concept 05: Universal Organizational Concepts
- Concept 06: What Does Knockout Give Us
- Concept 07: Bindings and Views in Knockout
- Concept 08: Knockout Views Quiz
- Concept 09: Models in Knockout
- Concept 10: Knockout Models Quiz
- Concept 11: Interview about Documentation
- Concept 12: Smarter Arrays
- Concept 13: Smart Models Work Differently
- Concept 14: Benefits of Smart Models
- Concept 15: Similarities between jQuery and KnockOut
- Concept 16: Building Something with Knockout
- Concept 17: Cat Clicker HTML and Bindings
- Concept 18: Computed Observables
- Concept 19: Computed Observables Quiz
- Concept 20: Review of Terms
- Concept 21: Computed Observables in Practice
- Concept 22: Add Cat Levels to Cat Clicker
- Concept 23: Show Cats With Control Structures
- Concept 24: Separating Out the Model
- Concept 25: 'with' and Binding Contexts
- Concept 26: How I Implemented "With"
- Concept 27: Getting Ready To Add More Cats
- Concept 28: Adding More Cats
-
Lesson 04: Learning a New Codebase
Learn how to work with new codebases and gain familiarity with the popular BackboneJS Framework.
- Concept 01: Interview: Gaining Context
- Concept 02: What's Next
- Concept 03: Exploring a New Codebase
- Concept 04: Codebase Quiz
- Concept 05: Getting the Big Picture of our Library
- Concept 06: Interview: Be Tofu
- Concept 07: Exploring a Codebase
- Concept 08: Modifying a Feature
- Concept 09: Adding Additional UI
- Concept 10: Adding a New Feature
- Concept 11: More Ideas
-
-
Module 03: Google Maps APIs
-
Lesson 01: Getting Started with the APIs
Set up your developer credentials and get started with the Google Maps APIs.
- Concept 01: Intro
- Concept 02: Your Project
- Concept 03: Your API Key
- Concept 04: The JavaScript API Overview
- Concept 05: Hello Map
- Concept 06: Making your Mark
- Concept 07: Window Shopping Part 1
- Concept 08: Window Shopping Part 2
- Concept 09: Markers Infowindows
- Concept 10: Being Stylish
- Concept 11: Styles
- Concept 12: Static Maps and Street View Imagery
- Concept 13: Imagery
- Concept 14: Pitch and Heading
- Concept 15: Library Cards
- Concept 16: Geometry and Visualization
- Concept 17: Drawing
- Concept 18: Drawing and Geometry
-
Lesson 02: Understanding API Services
Explore the location services available in the Google Maps APIs, including the Geocoding, Elevation, and Directions APIs.
- Concept 01: Web Services and Geocoding Part 1
- Concept 02: Web Services and Geocoding Part 2
- Concept 03: Geocoding Requests and Responses
- Concept 04: Status
- Concept 05: Geocoding Requests and Responses
- Concept 06: Geocoding in the App
- Concept 07: Interpreting Geocoding Responses
- Concept 08: No Mountain High Enough - Elevation API
- Concept 09: My Commute - Distance Matrix API Part 1
- Concept 10: My Commute - Distance Matrix API Part 2
- Concept 11: I want to ride my bicycle
- Concept 12: My Commute - Directions API
- Concept 13: Displaying Routes Directions Service
- Concept 14: From me to you
- Concept 15: Distance Matrix and Directions Specifics
- Concept 16: The Long and Winding Roads Roads API
- Concept 17: The Roads Less Travelled
- Concept 18: Speed Limits Request
- Concept 19: Roads API
- Concept 20: Faster is Better - Place Autocomplete 1
- Concept 21: Faster is Better - Place Autocomplete 2
- Concept 22: Devil in the Details - Places Details
- Concept 23: Places I Remember
- Concept 24: Here, There, and Everywhere
- Concept 25: Across the Universe TimeZone API
- Concept 26: Time after Time
- Concept 27: I am here, oh wait, where am I?
- Concept 28: The Journey so Far
-
Lesson 03: Using the APIs in Practice
Learn the practical details you need to know to use the Google Maps APIs in the real world.
-
Lesson 04: Neighborhood Map
You will develop a single-page application featuring a map of your neighborhood or a neighborhood you would like to visit. You will then add additional functionality to this application, including: map markers to identify popular locations or places you'd like to visit, a search function to easily discover these locations, and a listview to support simple browsing of all locations. You will then research and implement third-party APIs that provide additional information about each of these locations (such as StreetView images, Wikipedia articles, Yelp reviews, etc).
-
Part 07 : Project - Linux Server Configuration
You will take a baseline installation of a Linux distribution on a virtual machine and prepare it to host your web applications, to include installing updates, securing it from a number of attack vectors and installing/configuring web and database servers.
-
Module 01: Configuring Linux Web Servers
-
Lesson 01: Intro to Linux
Gain an understanding of the Linux operating system and how it differs from other operating systems you may have experienced in the past.
- Concept 01: Introduction
- Concept 02: What is Linux
- Concept 03: Free as in Beer
- Concept 04: Intro to Distributions
- Concept 05: Getting Started with Vagrant
- Concept 06: Linux Distribution Comparison
- Concept 07: Vagrant Commands
- Concept 08: This is Not Your Computer
- Concept 09: Where are we
- Concept 10: Your Home Directory
- Concept 11: Users Home Directory
- Concept 12: Other Important Directories
- Concept 13: Traversing the File System
- Concept 14: Understanding $PATH
- Concept 15: Outro
-
Lesson 02: Linux Security
Dive deep into Linux Security to ensure your service remains stable and free from attackers.
- Concept 01: Intro to Linux Security
- Concept 02: The Rule of Least Privilege
- Concept 03: Becoming a Super User
- Concept 04: sudo vs. su
- Concept 05: Package Source Lists
- Concept 06: Updating Available Package Lists
- Concept 07: Upgrading Installed Packages
- Concept 08: Other Package Related Tasks
- Concept 09: Discovering Packages
- Concept 10: Using Package Search
- Concept 11: Using Finger
- Concept 12: Introduction to /etc/passwd
- Concept 13: Reading /etc/passwd Entries
- Concept 14: User Account Info
- Concept 15: Introduction to User Management
- Concept 16: Creating a New User
- Concept 17: Connecting as the New User
- Concept 18: Introduction to etc sudoers
- Concept 19: Giving Sudo Access
- Concept 20: Resetting Passwords
- Concept 21: Another Authentication Method
- Concept 22: Public Key Encryption
- Concept 23: Generating Key Pairs
- Concept 24: Supported Key Types
- Concept 25: Installing a Public Key
- Concept 26: Forcing Key Based Authentication
- Concept 27: Introduction to File Permissions
- Concept 28: Owners and Groups
- Concept 29: Identifying Owners and Groups
- Concept 30: Octal Permissions [missing dialogue]
- Concept 31: Octal File Permissions
- Concept 32: chgrp and chown
- Concept 33: Intro to Ports
- Concept 34: Default Ports for Popular Services
- Concept 35: Intro to Firewalls
- Concept 36: Intro to UFW
- Concept 37: Configuring Ports in UFW
- Concept 38: Conclusion
-
Lesson 03: Web Application Servers
Install all of the required software to turn your Linux server into a full-fledged web application server and host your very own application!
-
-
Module 02: Project: Linux Server Configuration
-
Lesson 01: Linux Server Configuration
You will take a baseline installation of a Linux distribution on a virtual machine and prepare it to host your web applications.
-
Part 08 (Elective): Elective Course: Intro to JavaScript
-
Module 01: Intro to JavaScript
-
Lesson 01: What is JavaScript?
Learn the history of JavaScript and start writing your code immediately using the JavaScript console.
-
Lesson 02: Data Types & Variables
Learn to represent real-world data using JavaScript variables, and distinguish between the different data types in the language.
- Concept 01: Intro to Data Types
- Concept 02: Numbers
- Concept 03: Comments
- Concept 04: Quiz: First Expression (2-1)
- Concept 05: Strings
- Concept 06: String Concatenation
- Concept 07: Variables
- Concept 08: Quiz: Converting Temperatures (2-2)
- Concept 09: String Index
- Concept 10: Escaping Strings
- Concept 11: Comparing Strings
- Concept 12: Quiz: Favorite Food (2-3)
- Concept 13: Quiz: String Equality for All (2-4)
- Concept 14: Quiz: All Tied Up (2-5)
- Concept 15: Quiz: Yosa Buson (2-6)
- Concept 16: Booleans
- Concept 17: Quiz: Facebook Post (2-7)
- Concept 18: Null, Undefined, and NaN
- Concept 19: Equality
- Concept 20: Quiz: Semicolons! (2-8)
- Concept 21: Quiz: What's my Name? (2-9)
- Concept 22: Quiz: Out to Dinner (2-10)
- Concept 23: Quiz: Mad Libs (2-11)
- Concept 24: Quiz: One Awesome Message (2-12)
- Concept 25: Lesson 2 Summary
-
Lesson 03: Conditionals
Learn how to add logic to your JavaScript programs using conditional statements.
- Concept 01: Intro to Conditionals
- Concept 02: Quiz: Flowcharts (3-1)
- Concept 03: Flowchart to Code
- Concept 04: If...Else Statements
- Concept 05: Else If Statements
- Concept 06: Quiz: Even or Odd (3-2)
- Concept 07: Quiz: Musical Groups (3-3)
- Concept 08: Quiz: Murder Mystery (3-4)
- Concept 09: More Complex Problems
- Concept 10: Logical Operators
- Concept 11: Logical AND and OR
- Concept 12: Quiz: Checking your Balance (3-5)
- Concept 13: Quiz: Ice Cream (3-6)
- Concept 14: Quiz: What do I Wear? (3-7)
- Concept 15: Advanced Conditionals
- Concept 16: Truthy and Falsy
- Concept 17: Ternary Operator
- Concept 18: Quiz: Navigating the Food Chain (3-8)
- Concept 19: Switch Statement
- Concept 20: Falling-through
- Concept 21: Quiz: Back to School (3-9)
- Concept 22: Lesson 3 Summary
-
Lesson 04: Loops
Harness the power of JavaScript loops to reduce code duplication and automate repetitive tasks.
- Concept 01: Intro to Loops
- Concept 02: While Loops
- Concept 03: Parts of a While Loop
- Concept 04: Quiz: JuliaJames (4-1)
- Concept 05: Quiz: 99 Bottles of Juice (4-2)
- Concept 06: Quiz: Countdown, Liftoff! (4-3)
- Concept 07: For Loops
- Concept 08: Parts of a For Loop
- Concept 09: Nested Loops
- Concept 10: Increment and Decrement
- Concept 11: Quiz: Changing the Loop (4-4)
- Concept 12: Quiz: Fix the Error 1 (4-5)
- Concept 13: Quiz: Fix the Error 2 (4-6)
- Concept 14: Quiz: Factorials! (4-7)
- Concept 15: Quiz: Find my Seat (4-8)
- Concept 16: Lesson 4 Summary
-
Lesson 05: Functions
Dive into the world of JavaScript functions. Learn to harness their power to streamline and organize your programs.
- Concept 01: Intro to Functions
- Concept 02: Function Example
- Concept 03: Declaring Functions
- Concept 04: Function Recap
- Concept 05: Quiz: Laugh it Off 1 (5-1)
- Concept 06: Quiz: Laugh it Off 2 (5-2)
- Concept 07: Return Values
- Concept 08: Using Return Values
- Concept 09: Scope
- Concept 10: Scope Example
- Concept 11: Shadowing
- Concept 12: Global Variables
- Concept 13: Scope Recap
- Concept 14: Hoisting
- Concept 15: Hoisting Recap
- Concept 16: Quiz: Build a Triangle (5-3)
- Concept 17: Function Expressions
- Concept 18: Patterns with Function Expressions
- Concept 19: Function Expression Recap
- Concept 20: Quiz: Laugh (5-4)
- Concept 21: Quiz: Cry (5-5)
- Concept 22: Quiz: Inline (5-6)
- Concept 23: Lesson 5 Summary
-
Lesson 06: Arrays
Learn how to use Arrays to store complex data in your JavaScript programs.
- Concept 01: Intro to Arrays
- Concept 02: Donuts to Code
- Concept 03: Creating an Array
- Concept 04: Accessing Array Elements
- Concept 05: Array Index
- Concept 06: Quiz: UdaciFamily (6-1)
- Concept 07: Quiz: Building the Crew (6-2)
- Concept 08: Quiz: The Price is Right (6-3)
- Concept 09: Array Properties and Methods
- Concept 10: Length
- Concept 11: Push
- Concept 12: Pop
- Concept 13: Splice
- Concept 14: Quiz: Colors of the Rainbow (6-4)
- Concept 15: Quiz: Quidditch Cup (6-5)
- Concept 16: Quiz: Joining the Crew (6-6)
- Concept 17: Quiz: Checking out the Docs (6-7)
- Concept 18: Array Loops
- Concept 19: The forEach Loop
- Concept 20: Quiz: Another Type of Loop (6-8)
- Concept 21: Map
- Concept 22: Quiz: I Got Bills (6-9)
- Concept 23: Arrays in Arrays
- Concept 24: 2D Donut Arrays
- Concept 25: Quiz: Nested Numbers (6-10)
- Concept 26: Lesson 6 Summary
-
Lesson 07: Objects
Meet the next JavaScript data structure: the Object. Learn to use it to store complex data alongside Arrays.
- Concept 01: Intro to Objects
- Concept 02: Objects in Code
- Concept 03: Quiz: Umbrella (7-1)
- Concept 04: Objects
- Concept 05: Object Literals
- Concept 06: Naming Conventions
- Concept 07: Summary of Objects
- Concept 08: Quiz: Menu Items (7-2)
- Concept 09: Quiz: Bank Accounts 1 (7-3)
- Concept 10: Quiz: Bank Accounts 2 (7-4)
- Concept 11: Quiz: Facebook Friends (7-5)
- Concept 12: Quiz: Donuts Revisited (7-6)
- Concept 13: Lesson 7 Summary
-
Part 09 (Elective): Elective Course: Networking for Web Developers
-
Module 01: Networking
-
Lesson 01: From Ping to HTTP
Start exploring the network using low-level command-line tools such as ping and netcat.
- Concept 01: Intro
- Concept 02: Setting Up For This Course
- Concept 03: Try some network things!
- Concept 04: ping 8.8.8.8
- Concept 05: Ping versus HTTP
- Concept 06: Write your own HTTP to Wikipedia
- Concept 07: printf and netcat
- Concept 08: What web server does Google use?
- Concept 09: printf and netcat illustrate layers
- Concept 10: Listen on a Port
- Concept 11: Waiting For Your Call
- Concept 12: Listening and Connecting
- Concept 13: Experiment with nc and HTTP
- Concept 14: Port Numbers
- Concept 15: Port Numbers Part Two
- Concept 16: One listening server per port
- Concept 17: Be a Web Server
- Concept 18: Outro
-
Lesson 02: Names and Addresses
Investigate the Domain Name System (DNS), which translates hostnames into IP addresses.
- Concept 01: Intro
- Concept 02: Ping a Hostname
- Concept 03: Intro to DNS
- Concept 04: The host command
- Concept 05: Dig into DNS Records
- Concept 06: Research DNS Record Types
- Concept 07: DNS is Distributed with Caching
- Concept 08: DNS and HTTP
- Concept 09: Subdomains and FQDNs
- Concept 10: Register a Domain
- Concept 11: 32 bits to 128 bits
- Concept 12: 8 Bits in a Byte
- Concept 13: Bits
- Concept 14: Bits in a Port Number
- Concept 15: Outro
-
Lesson 03: Addressing and Networks
Investigate the structure and use of Internet addresses, including network blocks, interfaces, network address translation (NAT), and IPv6.
- Concept 01: Intro
- Concept 02: Special Addresses
- Concept 03: Reserved IP addresses
- Concept 04: How Many Addresses
- Concept 05: Netblocks and Subnets
- Concept 06: Subnet Masks
- Concept 07: Stanford's /14
- Concept 08: Not Enough Addresses
- Concept 09: The Host is a Lie
- Concept 10: Interfaces Quiz
- Concept 11: Routers and Default Gateways
- Concept 12: Default Gateway Quiz
- Concept 13: NAT
- Concept 14: Private and Public
- Concept 15: IPv6
- Concept 16: IPv6 Quiz
- Concept 17: Outro
-
Lesson 04: Protocol Layers
Use tcpdump to examine packets that make up the requests and responses for three protocols: ping, DNS, and HTTP.
- Concept 01: Intro: HTTP on TCP on IP
- Concept 02: The Protocol Stack
- Concept 03: Watching Ping and DNS in tcpdump
- Concept 04: Watching HTTP in tcpdump
- Concept 05: Analyzing the tcpdump data
- Concept 06: tcpdump packet quiz
- Concept 07: Sequence Diagrams
- Concept 08: Connection Establishment
- Concept 09: Buffering
- Concept 10: TCP Flags
- Concept 11: Why do packets drop
- Concept 12: TCP Errors
- Concept 13: Timeouts
- Concept 14: Errors Quiz
- Concept 15: Outro
-
Lesson 05: Big Networks
Learn more about bandwidth, latency, filtering, and other properties that matter when users are accessing your application over the Internet.
- Concept 01: Intro: Slow Sucks!
- Concept 02: Hops: Not Just for Beer
- Concept 03: The Story about Ping
- Concept 04: The Story about Traceroute
- Concept 05: Bandwidth across Networks
- Concept 06: What is Fast Anyway
- Concept 07: Bandwidth-Delay Product
- Concept 08: Congestion
- Concept 09: Middleboxes 1: Firewalls & Filtering
- Concept 10: What can a filter do?
- Concept 11: Middleboxes 2: Proxies & NAT
- Concept 12: Users behind a NAT
- Concept 13: Outro
-
Part 10 (Elective): Elective Material: Full Stack Webcasts
This is an archive of webcasts related to the Full Stack curriculum. Everything here is entirely optional!
-
Module 01: Webcasts
-
Lesson 01: Webcast Archive
This is an archive of webcasts related to the Full Stack curriculum. Everything here is entirely optional!
-
Part 11 (Career): Career: Job Search Strategies
-
Module 01: Conduct a Job Search
-
Lesson 01: Conduct a Job Search
Learn how to search for jobs effectively through industry research, and targeting your application to a specific role.
-
-
Module 02: Refine Your Resume
-
Lesson 01: Refine Your Entry-Level Resume
Receive a personalized review of your resume. This resume review is best suited for applicants who have 0-3 years of work experience in any industry.
-
Lesson 02: Refine Your Career Change Resume
Receive a personalized review of your resume. This resume review is best suited for applicants who have 3+ years of work experience in an unrelated field.
-
Lesson 03: Refine Your Prior Industry Experience Resume
Receive a personalized review of your resume. This resume review is best suited for applicants who have 3+ years of work experience in a related field.
Project Description - Resume Review Project (Prior Industry Experience)
Project Rubric - Resume Review Project (Prior Industry Experience)
- Concept 01: Convey Your Skills Concisely
- Concept 02: Effective Resume Components
- Concept 03: Resume Structure
- Concept 04: Describe Your Work Experiences
- Concept 05: Resume Reflection
- Concept 06: Resume Review
- Concept 07: Resume Review (Prior Industry Experience)
- Concept 08: Resources in Your Career Portal
-
-
Module 03: Write an Effective Cover Letter
-
Lesson 01: Craft Your Cover Letter
Get a personalized review of your cover letter. A successful cover letter will convey your enthusiasm, specific technical qualifications, and communication skills applicable to the position.
-
Part 12 (Career): Career: Networking
-
Module 01: Develop Your Personal Brand
-
Lesson 01: Develop Your Personal Brand
In this lesson, learn how to tell your unique story in a succinct and professional way. Communicate to employers that you know how to solve problems, overcome challenges, and achieve results.
-
Lesson 02: LinkedIn Review
Optimize your LinkedIn profile to show up in recruiter searches, build your network, and attract employers. Learn to read your LinkedIn profile through the lens of a recruiter or hiring manager.
-
-
Module 02: GitHub Profile Review
-
Lesson 01: GitHub Review
Review how your GitHub profile, projects, and code represent you as a potential job candidate. Learn to assess your GitHub profile through the eyes of a recruiter or hiring manager.
- Concept 01: Introduction
- Concept 02: GitHub profile important items
- Concept 03: Good GitHub repository
- Concept 04: Interview with Art - Part 1
- Concept 05: Identify fixes for example “bad” profile
- Concept 06: Quick Fixes #1
- Concept 07: Quick Fixes #2
- Concept 08: Writing READMEs with Walter
- Concept 09: Interview with Art - Part 2
- Concept 10: Commit messages best practices
- Concept 11: Reflect on your commit messages
- Concept 12: Participating in open source projects
- Concept 13: Interview with Art - Part 3
- Concept 14: Participating in open source projects 2
- Concept 15: Starring interesting repositories
- Concept 16: Outro
- Concept 17: Resources in Your Career Portal
-
Part 13 (Career): Career: Full Stack Interview Practice
-
Module 01: Interview Practice (Full Stack)
-
Lesson 01: Ace Your Interview
Learn strategies to prepare yourself for an interview.
-
Lesson 02: Practice Behavioral Questions
Practice answering behavioral questions and evaluate sample responses.
- Concept 01: Introduction
- Concept 02: Self-Practice: Behavioral Questions
- Concept 03: Analyzing Behavioral Answers
- Concept 04: Time When You Showed Initiative?
- Concept 05: What Motivates You at the Workplace?
- Concept 06: A Problem and How You Dealt With It?
- Concept 07: What Do You Know About the Company?
- Concept 08: Time When You Dealt With Failure?
-
Lesson 03: Interview Fails
Some real-life examples of interviews that didn't go as expected - it happens all the time!
-
Lesson 04: Land a Job Offer
You've practiced a lot for the interview by now. Continue practicing, and you'll ace the interview!
-
Lesson 05: Interview Practice
Walk through an example Full Stack interview and find out how you can improve!
- Concept 01: Analyzing an Interview
- Concept 02: Is it a Palindrome?
- Concept 03: What happens when you go to google.com?
- Concept 04: Describe an xsrf attack
- Concept 05: Find 2 elements that add up to K
- Concept 06: Overall Feedback
- Concept 07: Keep Practicing!
- Concept 08: Resources in Your Career Portal
-
-
Module 02: Data Structures & Algorithms with Python
-
Lesson 01: Introduction and Efficiency
Begin the section on data structures and algorithms, including Python and efficiency practice.
- Concept 01: Course Introduction
- Concept 02: Course Outline
- Concept 03: Course Expectations
- Concept 04: Syntax
- Concept 05: Python Practice
- Concept 06: Python: The Basics
- Concept 07: Efficiency
- Concept 08: Notation Intro
- Concept 09: Notation Continued
- Concept 10: Worst Case and Approximation
- Concept 11: Efficiency Practice
-
Lesson 02: List-Based Collections
Learn the definition of a list in computer science, and see definitions and examples of list-based data structures, arrays, linked lists, stacks, and queues.
- Concept 01: Welcome to Collections
- Concept 02: Lists
- Concept 03: Arrays
- Concept 04: Python Lists
- Concept 05: Linked Lists
- Concept 06: Linked Lists in Depth
- Concept 07: Linked List Practice
- Concept 08: Stacks
- Concept 09: Stacks Details
- Concept 10: Stack Practice
- Concept 11: Queues
- Concept 12: Queue Practice
-
Lesson 03: Searching and Sorting
Explore how to search and sort with list-based data structures, including binary search and bubble, merge, and quick sort. Learn how to use recursion.
- Concept 01: Binary Search
- Concept 02: Efficiency of Binary Search
- Concept 03: Binary Search Practice
- Concept 04: Recursion
- Concept 05: Recursion Practice
- Concept 06: Intro to Sorting
- Concept 07: Bubble Sort
- Concept 08: Efficiency of Bubble Sort
- Concept 09: Bubble Sort Practice
- Concept 10: Merge Sort
- Concept 11: Efficiency of Merge Sort
- Concept 12: Merge Sort Practice
- Concept 13: Quick Sort
- Concept 14: Efficiency of Quick Sort
- Concept 15: Quick Sort Practice
-
Lesson 04: Maps and Hashing
Understand the concepts of sets, maps (dictionaries), and hashing. Examine common problems and approaches to hashing, and practice with examples.
-
Lesson 05: Trees
Learn the concepts and terminology associated with tree data structures. Investigate tree types, such as binary search trees, heaps, and self-balancing trees.
- Concept 01: Trees
- Concept 02: Tree Basics
- Concept 03: Tree Terminology
- Concept 04: Tree Practice
- Concept 05: Tree Traversal
- Concept 06: Depth-First Traversals
- Concept 07: Tree Traversal Practice
- Concept 08: Search and Delete
- Concept 09: Insert
- Concept 10: Binary Search Trees
- Concept 11: Binary Tree Practice
- Concept 12: BSTs
- Concept 13: BST Complications
- Concept 14: BST Practice
- Concept 15: Heaps
- Concept 16: Heapify
- Concept 17: Heap Implementation
- Concept 18: Self-Balancing Trees
- Concept 19: Red-Black Trees - Insertion
- Concept 20: Tree Rotations
-
Lesson 06: Graphs
Examine the theoretical concept of a graph and understand common graph terms, coded representations, properties, traversals, and paths.
- Concept 01: Graph Introduction
- Concept 02: What Is a Graph?
- Concept 03: Directions and Cycles
- Concept 04: Connectivity
- Concept 05: Graph Practice
- Concept 06: Graph Representations
- Concept 07: Adjacency Matrices
- Concept 08: Graph Representation Practice
- Concept 09: Graph Traversal
- Concept 10: DFS
- Concept 11: BFS
- Concept 12: Graph Traversal Practice
- Concept 13: Eulerian Path
-
Lesson 07: Case Studies in Algorithms
Explore famous computer science problems, specifically the Shortest Path Problem, the Knapsack Problem, and the Traveling Salesman Problem.
-
Lesson 08: Technical Interview - Python
Practice with five technical interviewing questions on topics discussed in the data structures and algorithms course and get a personalized review on both your code and solutions.
- Concept 01: Interview Introduction
- Concept 02: Clarifying the Question
- Concept 03: Confirming Inputs
- Concept 04: Test Cases
- Concept 05: Brainstorming
- Concept 06: Runtime Analysis
- Concept 07: Coding
- Concept 08: Coding 2
- Concept 09: Debugging
- Concept 10: Interview Wrap-Up
- Concept 11: Time for Live Practice with Pramp
- Concept 12: Next Steps
- Concept 13: Resources in Your Career Portal
-